Microsoft is working on a Domain Specific Language (DSL) for abstracting the Azure ARM templates, https://github.com/Azure/bicep, Although the language is still in its infancy, it is a very useful language for generating ARM templates without the need to learn the complexities required. Furthermore, it allows reusable code to be implemented using modules.
Following some work that was done in the past with Terraform, which still required ARM templates to support it, it was imperative to look at Bicep as a supporting tool. To this regard, a simple Echo API used logic apps, which stores the responses in a blob was used as an experimentation playground.
Setting up the Datalake storage account
Before building the Logic App, which will require more knowledge of ARM template structure, a simple resource will be created. What can be simpler than a Storage Account.
To create a Storage Account using a minimalist ARM template, one would need to construct the following JSON:
{
"type": "Microsoft.Storage/storageAccounts",
"apiVersion": "2020-08-01-preview",
"name": "[parameters('storageAccounts_name')]",
"location": "[resourceGroup().location]",
"kind": "StorageV2",
"sku": {
"name": "Standard_LRS",
"tier": "Standard"
},
"properties": {
"supportsHttpsTrafficOnly": true,
"isHnsEnabled": true,
"minimumTlsVersion": "TLS1_2",
"accessTier": "Hot"
}
}
Translating the ARM Template above into Bicep, one will end up producing the following script:
resource storage_example 'Microsoft.Storage/storageAccounts@2020-08-01-preview' = {
name: 'storexampleweeu001'
location: resourceGroup().location
kind: 'StorageV2'
sku: {
name: 'Standard_LRS'
tier: 'Standard'
}
properties: {
supportsHttpsTrafficOnly: true
isHnsEnabled: true
minimumTlsVersion: 'TLS1_2'
accessTier: 'Hot'
}
}
It is immediately evident the similarity between the two scripts. First the type and the apiVersion are defined in the Bicep template to define what type of resource is required, Following that the template is very similar. Some of the minor noticeable differences are:
- Line 3 in the Bicep template makes it easier to determine that we are using a function to retrieve the hosting resource group location. While looking at the equivalent line in the ARM template, line 5, the first impression is that it is a normal string .
- Another noticeable difference is that strings in Bicep templates make use of single quotes, instead of the double quotes.
Other than the 2 differences mentioned above, the structure and the details are exactly the same.
Setting up the Blob storage for storing the data
Once the Storage Account has been created, a blob container or in this case a Datalake V2 container needs to initialised to host the blobs created by the Logic App.
The ARM template to create a blob container is as follows:
{ "type": "Microsoft.Storage/storageAccounts/blobServices/containers", "apiVersion": "2020-08-01-preview", "name": "[format('{0}/default/logicappstore', parameters('storageAccounts_name'))]", "dependsOn": [ "[resourceId('Microsoft.Storage/storageAccounts', parameters('storageAccounts_name')))]" ] }
Here the ARM template starts getting a little tougher to understand for beginners. In line 4, the name is dynamically created, and although the container will be named ‘logicappstore‘ it needs to be referenced as a folder structure with the Storage Account name being the root location. Furthermore, the line is making use of the string format function to construct the name. Finally in line 6, the resourceId()
function is introduced to fetch the unique identifier of the storage account, which is normally a subscription based path. However during ARM template coding the Id of the storage account might not be known, although one can try to guess.
Using Bicep to create the blob container, the first benefits of the language can start to emerge.
resource storage_blob_container 'Microsoft.Storage/storageAccounts/blobServices/containers@2020-08-01-preview' = {
name: '${storage_example.name}/default/logicappstore'
}
The first noticeable difference is the lack of need to define the depends on section. The depends on section in the ARM template will be used automatically added by the Bicep engine. The second difference is the way the name field is defined. In the Bicep language instead of using the format()
function, which is still possible the string interpolation is used. This makes the field value more readable. Finally, Bicep is able to refer to the name of the storage account by using the resource reference name provided as a second parameter to the resource command and accessing the name property.
Setting up API connection to the Azure Blob Storage
The logic app will require a connection to the Azure blob storage created earlier.
resource logic_app_blob_connection 'Microsoft.Web/connections@2016-06-01' = {
name: 'api-example-weeu-001'
location: resourceGroup().location
properties: {
displayName: 'api-example-weeu-001'
parameterValues: {
accountName: storage_example.name
accessKey: listKeys(storage_example.id, '2019-06-01').keys[0].value
}
api: {
id: concat('/subscriptions/', subscription().subscriptionId, '/providers/Microsoft.Web/locations/', resourceGroup().location, '/managedApis/azureblob')
}
}
}
Notice the use of listKeys
and concat
as functions within the Bicep language. This makes the code look more like the traditional programming language than the ARM template string format.
Setting up the Logic App
Now that the background work has been scripted, it is time to build the logic app ARM template.
resource logic_app_example 'Microsoft.Logic/workflows@2019-05-01' = {
name: 'logic-example-weeu-001'
location: resourceGroup().location
properties: {
definition: {
'$schema': 'https://schema.management.azure.com/providers/Microsoft.Logic/schemas/2016-06-01/workflowdefinition.json#'
triggers: {
'http_request': {
type: 'request'
kind: 'http'
inputs: {
schema: {
'$schema': 'http://json-schema.org/draft-04/schema#'
type: 'object'
properties: {
hello: {
type: 'string'
}
}
required: [
'hello'
]
}
}
operationOptions: 'EnableSchemaValidation'
}
}
actions: {
'HTTP_Response': {
type: 'Response'
inputs: {
...
}
runAfter: {
'Create_Blob': [
'Succeeded'
]
}
}
'Create_Blob': {
inputs: {
...
}
runAfter: {}
runtimeConfiguration: {
contentTransfer: {
transferMode: 'Chunked'
}
}
type: 'ApiConnection'
}
}
parameters: {
'$connections': {
defaultValue: {}
type: 'Object'
}
}
}
parameters: {
'$connections': {
value: {
azureblob: {
connectionId: logic_app_blob_connection.id
connectionName: logic_app_blob_connection.name
id: concat('/subscriptions/', subscription().subscriptionId, '/providers/Microsoft.Web/locations/', resourceGroup().location, '/managedApis/azureblob')
}
}
}
}
}
}
Once the script is complete, it needs to be built to generate the Incremental ARM template. Finally the ARM template can be deployed to a resource group.
Testing the ECHO API
Using Postman the Logic App can be called and tested.
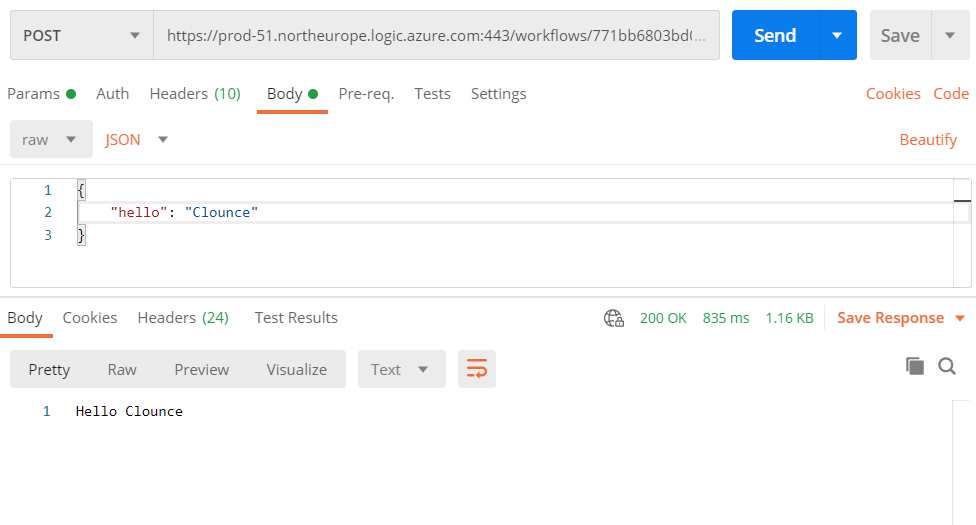
The Logic App also created a blob in the Storage Account using the timestamp as the blob name.
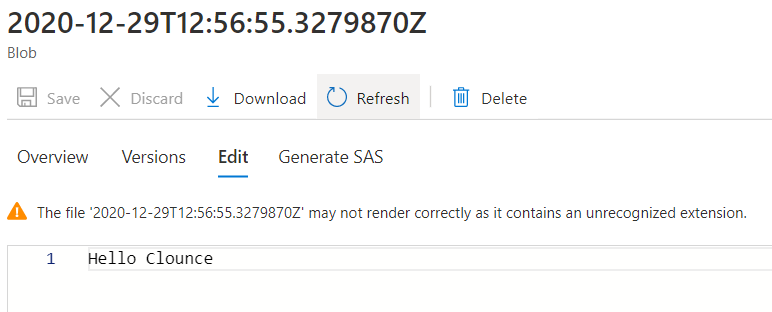
The code for this example can be found at https://github.com/kdemanuele/bicep-echo-api-logic-app