Recently Clounce was browsing some C# code and noticed that sometimes junior developers use throw exception
rather than simply throw
in try..catch
clauses.
Let’s see an example and then examine the results. Consider this piece of code:
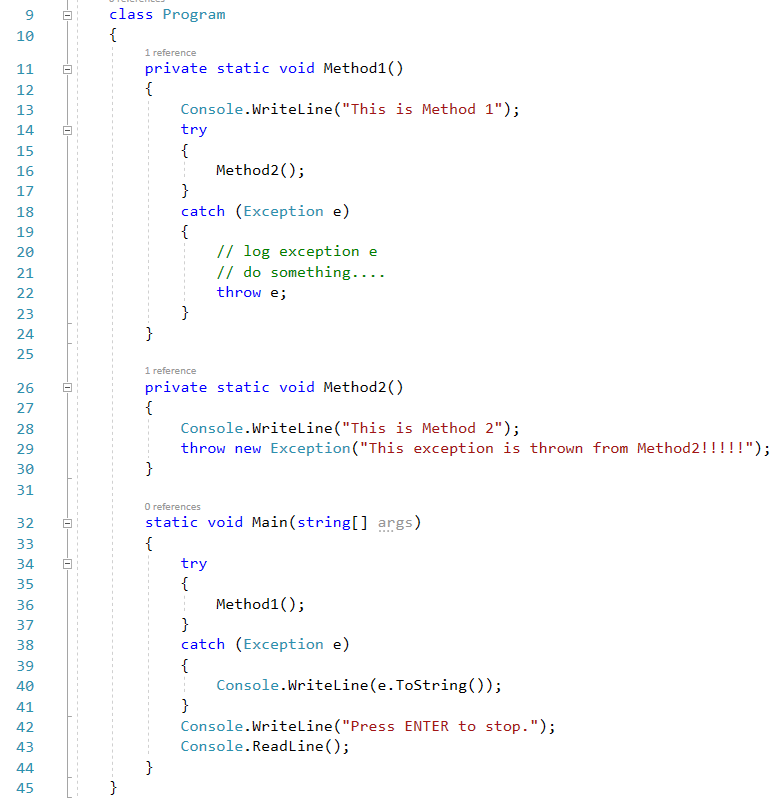
Of particular importance is the catch
block in Method1()
at line 22. In this block, the developer is catching the exception thrown by Method2()
in line 29 and throw it back to the Main()
method. This seems OK, but there is an issue with it. Let’s examine the stack trace printed to the console by line 40:
This is Method 1
This is Method 2
System.Exception: This exception is thrown from Method2!!!!!
at ThrowVsThrowEx.Program.Method1() in …\Program.cs:line 22
at ThrowVsThrowEx.Program.Main(String[] args) in …\Program.cs:line 36
Press ENTER to stop.
Note that the stack trace above tells us that the exception was raised from line 22, when in fact, it is initially raised by line 29! Why is it so? This is because line 22 is actually throwing exception e
again and resetting the stack trace. If we want to bubble up the exception caught in line 22, then we need to update it to read throw;
.
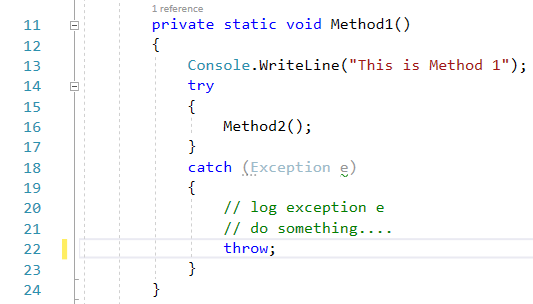
Running our program again will now print the correct stack trace:
This is Method 1
This is Method 2
System.Exception: This exception is thrown from Method2!!!!!
at ThrowVsThrowEx.Program.Method2() in …\Program.cs:line 29
at ThrowVsThrowEx.Program.Method1() in …\Program.cs:line 22
at ThrowVsThrowEx.Program.Main(String[] args) in …\Program.cs:line 36
Press ENTER to stop.
Note that our stack trace now shows line 29 as the cause of our exception. It is a subtle difference in the code but can saves us time troubleshooting code issues.
Code is available for download from https://github.com/jod75/ThrowVsThrowEx. Enjoy!